Java Programming - Beginner Level
Preference | Dates | Timing | Location | Registration Fees |
---|---|---|---|---|
Instructor-Led Training (In-Person and Live Webinars) |
6, 9, 13, 16, 20, 23, 27, 30 November 2023 | Mon., Thu.: 11:00AM - 1:30PM or 7:00PM - 9:30PM | Dubai Knowledge Park | 1225 USD |
Certification Exam | 4 December 2023 | Monday: 11:00 AM - 1:00 PM or 7:00 PM - 9:00 PM | Dubai Knowledge Park | Included |
Course Description
The Java Programming – Beginner Level course provides students, especially those with little or no prior programming experience, with an introduction to the world of Java programming. This course demonstrates the importance of object-oriented programming and the intricacies of the Java language, empowering students to build simple Java applications. Offering a comprehensive understanding of the Java programming language, students will emerge from this course ready for further exploration and growth. This program prominently features the Java Platform, Standard Edition 21 (Java SE 21). Upon successful completion of this program, the participants will earn a KHDA-Accredited Certificate.
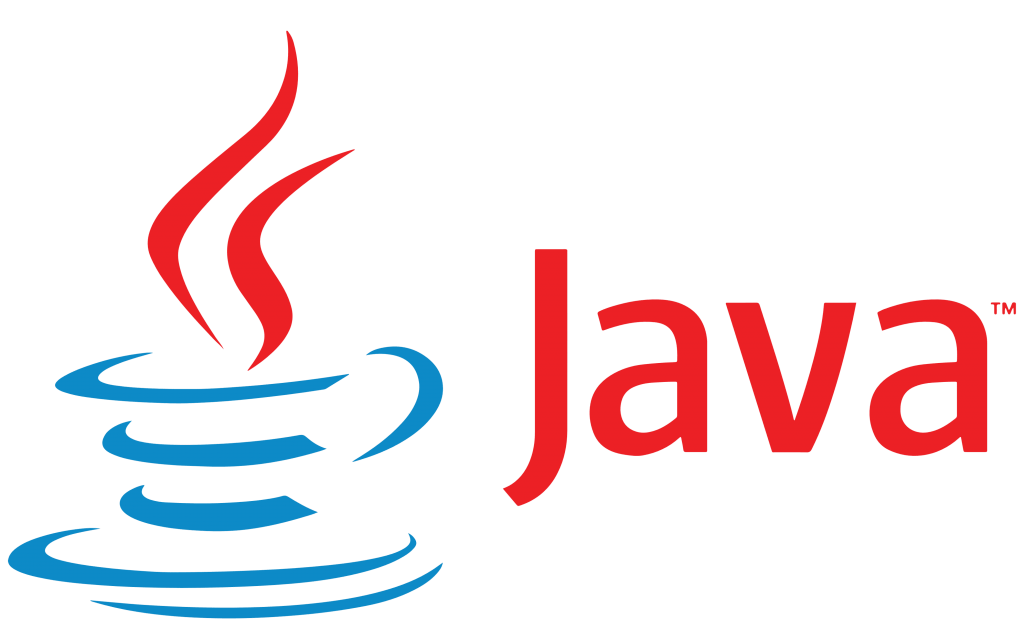
Course Outline
Audience
Prerequisites
Learning Outcomes
Course Outline
Unit 1 – Introducing the Java Technology
- Relating Java to Other Languages.
- Setting Up the Java Environment: Download, install, and configure.
- Exploring Java Technologies: Understand Java SE, Spring Boot, and JavaFX.
- Java’s Key Features: Discuss the core features and advantages of using Java.
- Using an Integrated Development Environment (IDE).
Unit 2 – Thinking in Objects
- Define the problem domain.
- Identify objects and recognize the criteria for defining them.
Unit 3 – Introducing the Java Language
- Defining classes.
- Identifying the components of a class.
- Creating and using a test class.
- Compiling and executing a test program.
Unit 4 – Working with Primitive Variables
- Declaring and initializing field variables
- Understanding primitive data types: integral, floating point, textual and logical.
- Declaring variables and assigning values.
- Using final keyword to create constants.
- Applying arithmetic operators to modify values.
Unit 5 – Working with Objects
- Declaring and initializing objects.
- Understanding how objects are stored in memory.
- Manipulating data using object references.
- Consulting Java SE Javadocs to research class methods.
- Working efficiently with String and StringBuilder classes.
Unit 6 – Using Operators and Decision Constructs
- Utilizing relational (e.g.,
==
,<
,>
,<=
,>=
) and conditional (e.g.,&&
,||
) operators. - Comparing strings using
equals()
and==
methods. - Evaluating conditions in a program and determining the algorithm’s flow.
- Constructing if and if/else statements for conditional logic.
- Nesting and chaining multiple conditional statements.
- Implementing switch statements for multi-branch decision-making.
Unit 7 – Creating and Using Arrays
- Declaring, instantiating, and initializing one-dimensional arrays.
- Declaring, instantiating, and initializing two-dimensional arrays.
- Processing arrays using for loops.
- Creating and initializing ArrayLists.
- Utilizing the import statement to incorporate existing Java APIs.
- Accessing values in both arrays and ArrayLists.
- Understanding and using the
args
array in the main method.
Unit 8 – Using Loop Constructs
- Creating and understanding while loops and nested while loops.
- Developing and using for loops.
- Iterating over ArrayLists using for loops.
- Constructing do-while loops.
- Grasping the concept of variable scope within loops and blocks.
Unit 9 – Working with Methods and Encapsulation
- Creating and invoking methods in Java.
- Passing arguments to methods and returning values.
- Defining static methods and static variables.
- Utilizing access modifiers for methods and variables.
- Overloading methods to provide multiple definitions.
- Constructing and understanding Java constructors.
- Implementing encapsulation to protect object integrity.
Unit 10 – Introducing Advanced Object Oriented Concepts
- Understanding and applying inheritance in Java.
- Exploring types of polymorphism: overloading, overriding, and dynamic binding.
- Working effectively with superclasses and subclasses.
- Integrating abstraction into analysis and design.
- Grasping the purpose and utility of Java interfaces.
- Designing and implementing interfaces in Java.
Unit 11 – Handling Errors
- Identifying and understanding the types of errors in Java.
- Grasping the varied kinds of Exceptions in Java.
- Utilizing Javadocs to research Exceptions thrown by foundational class methods.
- Implementing code to effectively handle Exceptions.
Unit 12 – The Big Picture
- Creating packages and deploying with JAR files
- Describing comprehensive Java applications that incorporate a middle tier and a database backend
Audience
This course is tailored for:
- Beginners to Programming: Individuals who are embarking on their journey in the world of programming and are looking for a structured pathway to understand the foundational elements of a powerful language like Java.
- Career Changers: Professionals from other fields who wish to transition into software development and see Java as an essential skill in the software development world.
- Students: Students from high school to college who wish to strengthen their programming capabilities for academic projects or future career prospects.
- Technical Managers: Those who wish to understand Java to better manage development teams or to communicate more effectively with technical stakeholders.
- Hobbyists and Enthusiasts: Individuals who have an innate curiosity about how software works and see Java as a stepping stone to creating personal projects or just understanding the tech world a bit better.
Prerequisites
Prerequisites
- A basic understanding of computer systems.
- Willingness to learn and problem-solve.
- No prior programming experience required.
Learning Outcomes
By the end of this course, learners will:
- Have a firm understanding of Java’s core concepts and how they interplay to create robust applications.
- Be equipped to write, debug, and deploy basic Java applications.
- Understand and apply object-oriented principles in Java.
- Be well-versed in handling errors and exceptions.
- Know the significance and usage of different Java technologies and tools, including IDEs.
- Be prepared to delve deeper into advanced Java topics or start building their Java-based applications.
Testimonials
Innosoft Gulf really gave me a head start for college. The teacher was amazing and I really learned a lot. I highly recommend the Python, Java and Machine Learning courses.
Oshin VatsDistinguished High School Student
I had a very positive experience taking a Java course with Ahmed El Koutbia (Instructor) at Innosoft Gulf. Ahmed El Koutbia is a former expert and architect of Java at Sun Microsystems.
The course was lab-based, and Ahmed explained every single line of code, enhancing my learning experience significantly.
Innosoft Gulf comes highly recommended if you wish to learn Java, Python, and AI. Ahmed provides direction, advice, and guides you through the learning path. Many thanks to Ahmed El Koutbia.
Omar IsmailMathematics Professor
The workshop on big data and machine learning was an excellent introduction to practitioners considering using data science. Ahmed demonstrated considerable teaching talent rooted in his long expertise with systems development.
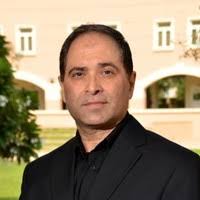
Very rewarding course. Rare to find a Deep learning course in Dubai that teaches concepts from scratch and provides practical applications. Will definitely recommend.
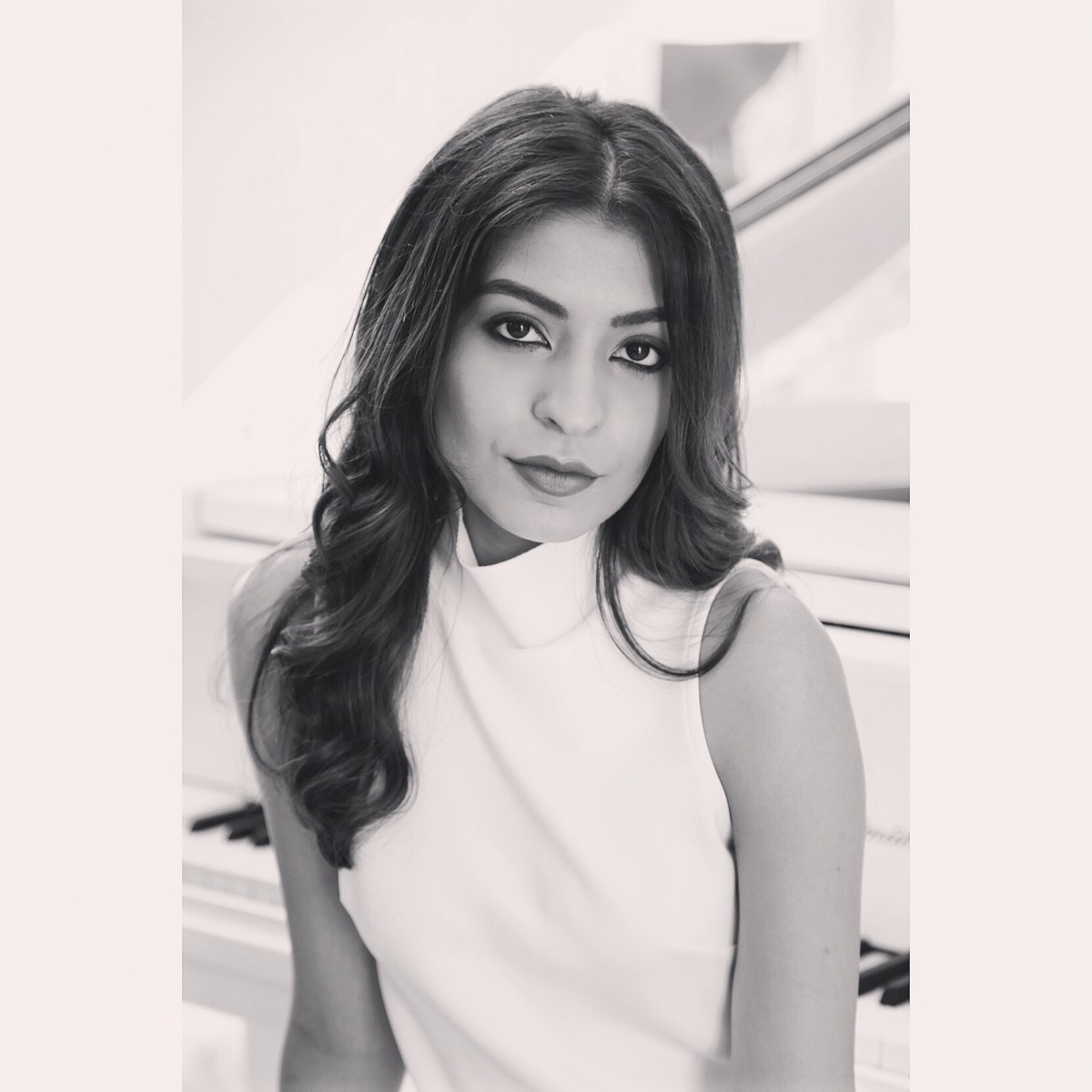
Innosoft Gulf Institute is educating students breaking and revolutionary techniques with focus on future trends in CIT industry. Mr. Ahmed is well updated on latest technologies related to Big Data, AI, Machine Learning, etc.
Rated as 5 star in terms of overall deliverance.
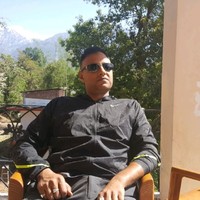
The most important thing is to be convinced of what you are studying. It's not just about teaching...
I'm taking four courses at Innosoft Gulf institute, and I think it's much better than my bachelor's degree.
Ahmad TahboubSenior Year Computer Science Student
I recently had the opportunity to participate in the Innosoft Gulf Python, Data Analytics, Data Visualization, and Machine Learning training program, and I am thrilled to share my experience with others. This program was nothing short of exceptional, and I have gained valuable knowledge and skills that have enhanced my career and personal growth.
Rakesh NairManager at a Pharmaceutical Company
It is stunning what I learned at the Python Engineering course for Financial Data Analysis and Algorithmic Trading. Thank you so much, I highly recommend this course.
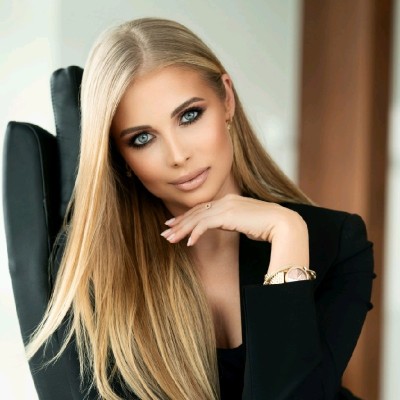